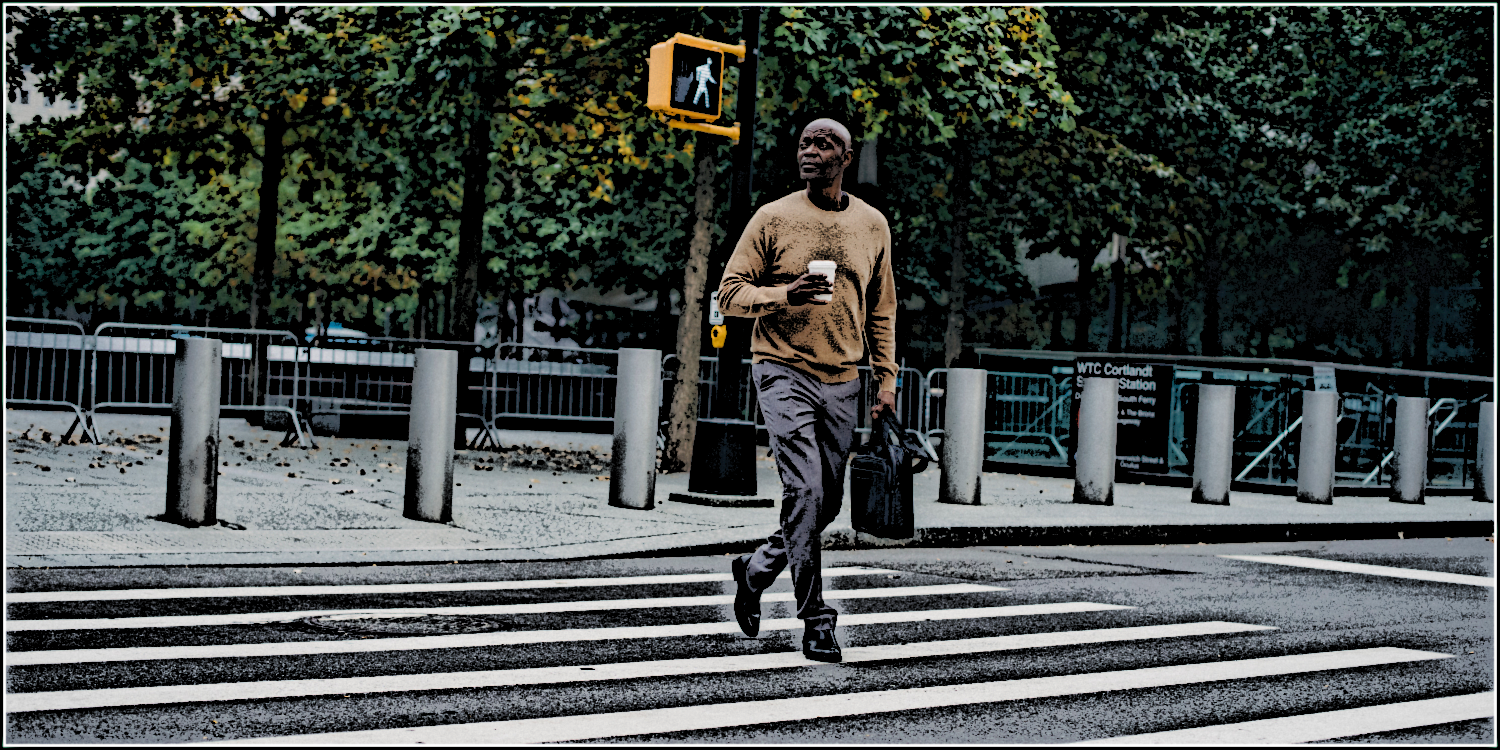
Contract by Design
Softlib
Runtime code safety and sanity checking

Abstract |
---|
The softlib JavaScript library has functions for type expectations, soft assertions, and logging to the terminal with stack trace. |
Motivation
Before typescript came on the scene this library was a good way to reliably verify that function inputs were in their expected form. It continues to be an essential library for software written in pure ECMAScript.
Features
The expect
function provides runtime type safety without the overhead of a precompiler. It provides a strong measure of safety during execution by providing an early warning system when things aren't proceeding as expected.
The aver
function is a soft assertion. When conditions should be true, an aver()
can alert the developer that things haven't lived up to their promise. This is done in a non-fatal way, allowing the condition to persist (even when the aver fails) letting the code deal with the condition using whatever coding paradigm is in force, such as a try/finally block or other exception/error handling techniques.
The terminal
function provides context sensitive logging. When reached via a browser, output is routed to the console as a warn() or error(). When reached via a node.js process, output is routed to stdout or stderr.
The vartype
function provides dynamic introspection of a variable's current type. It goes beyond ECMAScript's native typeof
and instanceof
keywords by inspecting the name of the variable's constructor.
The StackTrace
class provides a stack trace for the other functions.
Installation
The softlib library may be installed directly from github or via NPM.
To add the library to a node.js project, use this command:
[user@host]# npm install softlib
import {expect} from 'softlib';
import {aver} from 'softlib';
import {terminal} from 'softlib';
import {vartype} from 'softlib';
To add the library to a browser project, use this command:
[user@host]# git clone https://github.com/readwritetools/softlib.git
import {expect} from '/softlib/expect.js';
import {aver} from '/softlib/aver.js';
import {terminal} from '/softlib/terminal.js';
import {vartype} from '/softlib/vartype.js';
Metadata
Module exports
ES modules | true |
Common JS | false |
Suitability
Browser | API |
node.js | API |
Availability
![]() | Documentation | Read Write Hub |
![]() | Source code | github |
![]() | Package installation | npm |
License
The softlib library is licensed under the MIT License.
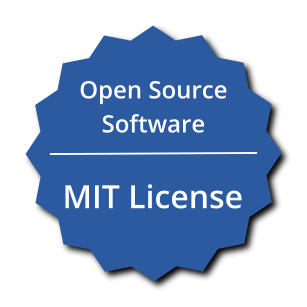
MIT License
Copyright © 2023 Read Write Tools.
Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.