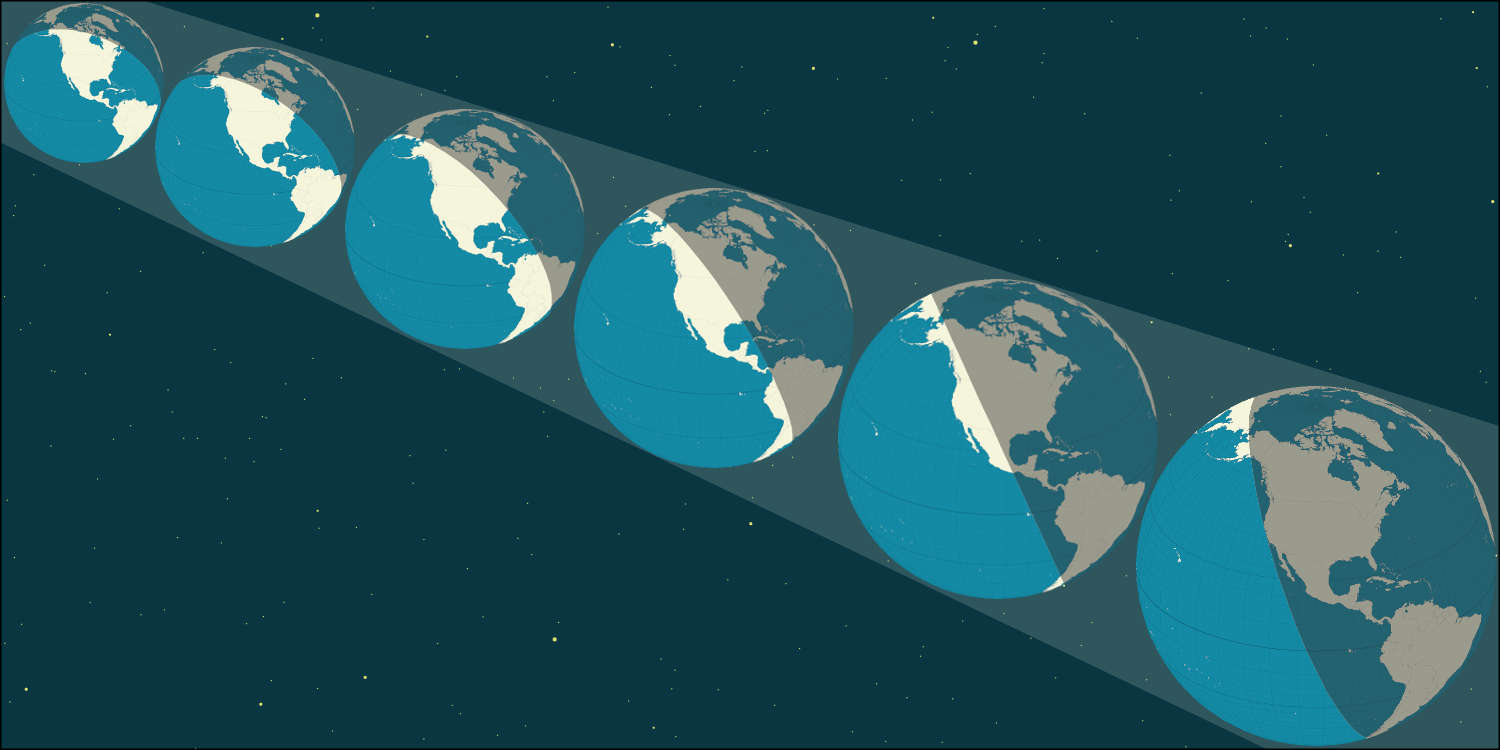
Premium DOM Component
Orthographic Earth
A world of possibilities

Abstract |
---|
The Orthographic Earth DOM component renders an interactive map of Earth where an entire hemisphere is visible. Its viewing angle can be rotated and tilted by the user to show Earth from any point of reference. Data can be added to the map using network-friendly quantized topoJSON layers. Data points can be linked to external databases to allow users to explore spatial-based thematic patterns. Data layers can be styled using a declarative language for visual style sheets. |
Background
All global maps suffer from distortion. This is because maps are a 2-dimensional projection of a 3-dimensional Earth. Attempts to display the entire world on a single rectangular canvas suffer more acutely than maps that are limited to just a portion of Earth at a time.
As an example, the Mercator projection exaggerates the size of the polar regions. This makes Canada, Greenland, and Russia appear much large than Africa, and turns Antarctica into an unrecognizable shape.
Another alternative — commonly used in thematic maps — is the equi-rectangular projection. It has less exaggerated areas than the Mercator projection, but still suffers because it plots each latitude without regard for the shorter longitudinal distances of the polar regions.
In contrast, the orthographic projection has faithful areas and angles near the point of perspective, with distortion occuring gradually, and only being noticable near the horizons. The primary drawback with orthographic projections in the past has been the fact that they only represent one-half of the world.
The Orthographic Earth DOM component provides an excellent alternative for cartographers who want to tell thematic stories on a global scale.
The Orthographic Earth component has these features:
- The component can be used within any HTML document.
- The drawing can be resized to fit the device's screen size, allowing it to be used on both small cell phones and large mega-pixel monitors.
- The component is self-contained, which allows for the possibility of having two or more Earth drawings appear side-by-side on the same page.
- The user interface supports both touchscreen and mouse gestures for scaling, translation, rotation and tilt.
- Users can locate and identify features by pointing to them.
- Webmasters can use the component's event-based interface to monitor user interactions, allowing synchronization with other parts of the HTML page.
- Features can be styled using a declarative visual stylesheet language.
Data layers can use sources with any of these supported formats. See GCS I/O for more about these formats. See Better Compression of GIS Features for optimization techniques.
- gfe - Geographic Feature Encoding
- ice - Indexed Coordinate Encoding
- tae - Topological Arc Encoding
- gfebin - Geographic Feature Encoding binary
- icebin - Indexed Coordinate Encoding binary
- taebin - Topological Arc Encoding binary
- geojson - RFC 7946
- topojson - (Specification)
In the wild
To see examples of this component in use, visit any of these:
full.earth | Polar views the world |
timezone.earth | Interactive map of world time |
simply.earth | Political geography of the world |
Installation
Prerequisites
The rwt-orthographic-earth DOM component works in any browser that supports modern W3C standards and the W3C Web Platform Incubator Community Group's importmap proposal. Chrome provides a good implementation of the importmap proposal, other browsers can use Guy Bedford's polyfill es-module-shims.
npm install es-module-shims
The rwt-orthographic-earth DOM component has three additional dependencies, also written by Read Write Tools:
- rwt-dockable-panels
- Dockable Panels DOM component.
- softlib
- Runtime code safety and sanity checking.
- GCS I/O
- Geographic Coordinate System I/O Library.
Download using NPM
Install the component and dependencies with these commands:
npm install rwt-orthographic-earth
npm install rwt-dockable-panels
npm install softlib
npm install gcsio
Important note: This DOM component uses Node.js and NPM and package.json
as a convenient distribution and installation mechanism. The DOM component itself does not need them.
Download using Github
If you prefer using Github, follow these steps:
- Create a
node_modules
directory in the root of your web project. - Clone the required repos into it using these commands:
git clone https://github.com/readwritetools/rwt-orthographic-earth.git
git clone https://github.com/readwritetools/rwt-dockable-panels.git
git clone https://github.com/readwritetools/softlib.git
git clone https://github.com/readwritetools/gcsio.git
Using the component
After installation, you need to add three things to your HTML page to make use of it:
- Add an
importmap
and ascript
tag to load the component and its dependencies: - Add the component tag somewhere on the page.
- For scripting purposes, apply an
id
attribute. - For WAI-ARIA accessibility apply a
role=contentinfo
attribute. - Listen for the component to be fully loaded before programmatically configuring its layers, stylesheets, and initial settings. Here's an example:
<script src='/node_modules/es-module-shims/dist/es-module-shims.js' async></script>
<script type=importmap-shim>
{
"imports": {
"gcsio/": "/node_modules/gcsio/",
"softlib/": "/node_modules/softlib/",
"rwt-dockable-panels/": "/node_modules/rwt-dockable-panels/"
}
}
</script>
<script src='/node_modules/rwt-orthographic-earth/rwt-orthographic-earth.js' type=module-shim></script>
<rwt-orthographic-earth id=earth1 role=contentinfo></rwt-orthographic-earth>
<script type=module-shim>
var earth1 = document.getElementById('earth1');
var promise = earth1.waitOnLoading();
promise.then(() => {
addLayers(earth1);
configure(earth1);
});
function addLayers(earth1) {
earth1.addVisualizationStyleSheet('/tess/earth1-styles.tess');
earth1.addMapItem({ layerType:'space', tessIdentifier: 'space', layerName: 'Space' });
earth1.addMapItem({ layerType:'sphere', tessIdentifier: 'sphere', layerName: 'Oceans' });
earth1.addMapItem({ layerType:'graticule', tessIdentifier: 'graticule-3-by-3',layerName: 'Graticule', meridianFrequency:3, parallelFrequency:3, drawToPoles:false });
earth1.addMapItem({ layerType:'gcs-package', tessIdentifier: 'land-110m', layerName: 'Land', url:'/natural-earth/land-110m.geojson', featureKey:'name' });
}
function configure(earth1) {
earth1.setLatitude(12.98);
earth1.setLongitude(77.58);
earth1.setRotationSpeed(-1);
}
</script>
Configure the Component
The component can be configured by adding any of these optional attributes to the <rwt-orthographic-earth>
element tag.
geolocation
Used to set the initial latitude, longitude and place of interest.-
''
[default] set initial longitude, latitude and place of interest to (0.0, 0.0) and honor explicit setTangentLongitude(), setTangentLatitude(), setPlaceOfInterest().'timezone'
use the browser's current time to determine the map's initial longitude.'auto'
use the browser's geolocation beacon to set initial longitude, latitude and place of interest.
menu-state
Used to set the initial state of the toolbar-
closed
[default] The toolbar menu should initially be collapsed.open
The toolbar menu should initially be expanded.
menu-corner
Used to position the toolbar's location relative to the component's canvas area.-
top-right
[default]top-left
bottom-right
bottom-left
panels
Declares the presence and order of menu panels.-
none
Hide the menu panel.interaction
Mouse gestures for manipulation and interaction.legend
Show the legend.season
Change the reference date.time-of-day
Change the time of day.equation-of-time
The variance between mean solar noon and actual solar noon.solar-events
The time of sunrise, solar noon, and sunset.geocentric-coords
The Earth's declination and right ascension for the current date and time.topocentric-coords
The position of the sun for the current location and time.point-of-reference
Change the longitude/latitude of the observation point.map-scale
Change the map's scale.space
Shift the space point of view.canvas
Shift the canvas origin.layers
Choose which layers to display.locate
Display longitude and latitude of mouse position.discover
Discover features a pointer position.identify
Identify underlying feature details.select
Highlight features by <Ctrl> 'n Click.distance
Display distances along great-circle arcs.time-lapse
Rotate the earth at a set speed.snapshot
Capture and download an image of the map.metadata
Show map citations, data sources, software version, and licensing links.symbol-specifier> Interactive manipulation of Thematic Earth Symbol Specifiers.
signals
Monitor system broadcasters and receivers.
Life-cycle events
The component issues life-cycle events.
component-loaded
- Sent when the component is fully loaded and ready to be used. As a convenience you can use the
waitOnLoading()
method which returns a promise that resolves when thecomponent-loaded
event is received. Call this asynchronously withawait
.
Metadata
Module exports
ES modules | true |
Common JS | false |
Suitability
Browser | API |
node.js | none |
Availability
![]() | Documentation | READ WRITE HUB |
![]() | Source code | github |
![]() | Component catalog | DOM COMPONENTS |
![]() | Package installation | npm |
![]() | Publication venue | READ WRITE STACK |
License
The rwt-orthographic-earth DOM component is not freeware. After evaluating it and before using it in a public-facing website, eBook, mobile app, or desktop application, you must obtain a license from Read Write Tools.
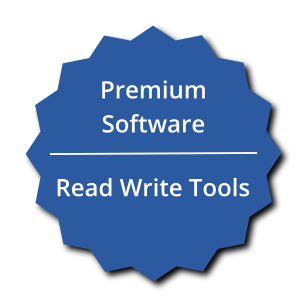
JavaScript Orthographic Earth Software License Agreement
Copyright © 2023 Read Write Tools.
- This Software License Agreement ("Agreement") is a legal contract between you and Read Write Tools ("RWT"). The "Materials" subject to this Agreement include the "JavaScript Orthographic Earth" software and associated documentation.
- By using these Materials, you agree to abide by the terms and conditions of this Agreement.
- The Materials are protected by United States copyright law, and international treaties on intellectual property rights. The Materials are licensed, not sold to you, and can only be used in accordance with the terms of this Agreement. RWT is and remains the owner of all titles, rights and interests in the Materials, and RWT reserves all rights not specifically granted under this Agreement.
- Subject to the terms of this Agreement, RWT hereby grants to you a limited, non-exclusive license to use the Materials subject to the following conditions:
- You may not distribute, publish, sub-license, sell, rent, or lease the Materials.
- You may not decompile or reverse engineer any source code included in the software.
- You may not modify or extend any source code included in the software.
- Your license to use the software is limited to the purpose for which it was originally intended, and does not include permission to extract, link to, or use parts on a separate basis.
- Each paid license allows use of the Materials under one "Fair Use Setting". Separate usage requires the purchase of a separate license. Fair Use Settings include, but are not limited to: eBooks, mobile apps, desktop applications and websites. The determination of a Fair Use Setting is made at the sole discretion of RWT. For example, and not by way of limitation, a Fair Use Setting may be one of these:
- An eBook published under a single title and author.
- A mobile app for distribution under a single app name.
- A desktop application published under a single application name.
- A website published under a single domain name. For this purpose, and by way of example, the domain names "alpha.example.com" and "beta.example.com" are considered to be separate websites.
- A load-balanced collection of web servers, used to provide access to a single website under a single domain name.
- THE MATERIALS ARE PROVIDED BY READ WRITE TOOLS "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL READ WRITE TOOLS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
- This license is effective for a one year period from the date of purchase or until terminated by you or Read Write Tools. Continued use, publication, or distribution of the Materials after the one year period, under any of this Agreement's Fair Use Settings, is subject to the renewal of this license.
- Products or services that you sell to third parties, during the valid license period of this Agreement and in compliance with the Fair Use Settings provision, may continue to be used by third parties after the effective period of your license.
- If you decide not to renew this license, you must remove the software from any eBook, mobile app, desktop application, web page or other product or service where it is being used.
- Without prejudice to any other rights, RWT may terminate your right to use the Materials if you fail to comply with the terms of this Agreement. In such event, you shall uninstall and delete all copies of the Materials.
- This Agreement is governed by and interpreted in accordance with the laws of the State of California. If for any reason a court of competent jurisdiction finds any provision of the Agreement to be unenforceable, that provision will be enforced to the maximum extent possible to effectuate the intent of the parties and the remainder of the Agreement shall continue in full force and effect.
Activation
To activate your license, copy the rwt-registration-keys.js
file to the root directory of your website, providing the customer-number
and access-key
sent to your email address, and replacing example.com
with your website's hostname. Follow this example:
export default [{
"product-key": "rwt-orthographic-earth",
"registration": "example.com",
"customer-number": "CN-xxx-yyyyy",
"access-key": "AK-xxx-yyyyy"
}]